In this article we will explore how to delete files using Python.
Table of Contents
- Introduction
- Sample files
- Delete a single file
- Delete multiple files
- Delete all files in a directory
- Delete entire directory
- Conclusion
Introduction
This article aims to provide a comprehensive guide on how to delete files using Python.
Whether you need to delete individual files, multiple files, or an entire directory, Python has the tools you need.
In this tutorial we will work with the os and shutil (“shell utility”) modules. os module provides a portable way of using operating system dependent functionality, and shutil module that provides a wide range of file operations. Both modules are a part of the standard library, so there is nothing additional to install.
Sample files
Here are the 3 sample files we will use in this tutorial:
and we will place them in the sample_files folder. Now your directory with the code file should look like this:
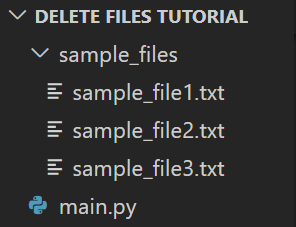
Delete a single file
In this section, we’ll explore how to delete a single file in a directory using Python.
This is one of the simplest operations when dealing with file management tasks, and Python makes it straightforward with the os module:
#Import the required dependency
import os
#Define path to folder where file is located
source_directory = 'sample_files/'
#Define the file name
file_name = 'sample_file1.txt'
#Get the relative full path to file
source_path = os.path.join(source_directory, file_name)
#Delete the file
os.remove(source_path)
print(f'File {source_path} deleted')
You should see the following message printed:
File sample_files/sample_file1.txt deleted
and you should see the file deleted from the directory:
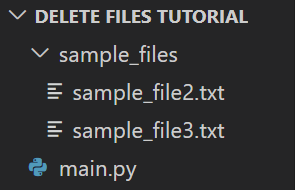
Delete multiple files
In this section, we’ll explore how to delete multiple files in a directory using Python.
The code will be 90% the same as in the previous section, except now we will iterate through a list of files and delete them one by one.
Note: In previous section we deleted one file (sample_file1.txt) from sample_files directory. Let’s recreate it back, so we have the original file structure:
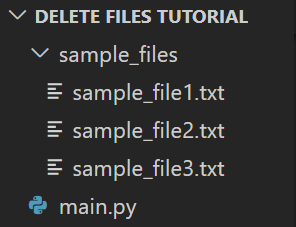
Now, to delete multiple files, we are going to create a list of file names that we want to delete and then use a loop to iterate through the list, generate file paths, and delete each file one at a time:
#Import the required dependency
import os
#Define path to folder where file is located
source_directory = 'sample_files/'
#Define the file name
file_names = ['sample_file1.txt', 'sample_file2.txt']
for file_name in file_names:
#Get the relative full path to file
source_path = os.path.join(source_directory, file_name)
#Delete the file
os.remove(source_path)
print(f'File {source_path} deleted')
You should see the following message printed:
File sample_files/sample_file1.txt deleted
File sample_files/sample_file2.txt deleted
and you should see the files deleted from the directory:
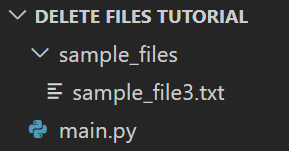
Delete all files in a directory
In this section, we’ll learn how to delete an all files from a directory using Python.
Note: In previous section we deleted multiple files (sample_file1.txt and sample_file2.txt) from sample_files directory. Let’s recreate them back, so we have the original file structure:
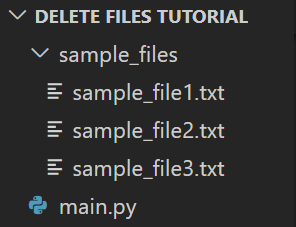
The code in this section will be 95% similar to the code in the previous section.
To delete all files in a directory we will need the list of all files and folders present in the source directory.
Using os.listdir() function we can generate a list of all files and folders, and then delete them one by one:
#Import the required dependency
import os
#Define path to folder where file is located
source_directory = 'sample_files/'
#Define the file names
file_names = os.listdir(source_directory)
#Iterate over files
for file_name in file_names:
#Get the relative full path to file
source_path = os.path.join(source_directory, file_name)
#Check if path represents a file
if os.path.isfile(source_path):
#Delete the file
os.remove(source_path)
print(f'File {source_path} deleted')
Note: the code above works for only deleting files in a given directory and it will not delete any folders (empty or not empty). This is why we are adding a check using os.path.isfile() function to make sure that a given path is a file (and not a folder).
You should see the following message printed:
File sample_files/sample_file1.txt deleted
File sample_files/sample_file2.txt deleted
File sample_files/sample_file3.txt deleted
and the directory should look empty:
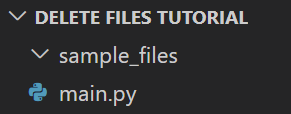
Note: main.py file wasn’t deleted as it is not in the sample_files directory.
Delete entire directory
In this section, we’ll learn how to delete an entire directory (including all of its subdirectories and files) using Python.
Note: In previous section we deleted all files (sample_file1.txt, sample_file2.txt, and sample_file3.txt) from sample_files directory. Let’s recreate them back, so we have the original file structure:
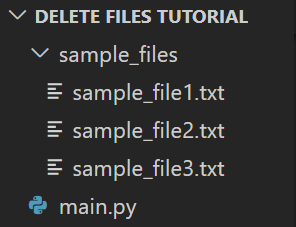
To delete an entire directory along with its contents, we will use the shutil.rmtree() function, which deletes an entire directory tree:
#Import the required dependency
import shutil
#Define path to directory
source_directory = 'sample_files/'
#Delete the directory
shutil.rmtree(source_directory)
print(f'Directory {source_directory} deleted')
You should see the following message printed:
Directory sample_files/ deleted
and you should see the directory deleted from the project directory:
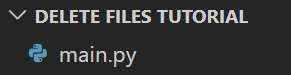
Note: main.py file wasn’t deleted as it is not in the sample_files directory.
Conclusion
In this article explored how to delete files and directories using Python with os and shutil modules.
Feel free to leave comments below if you have any questions or have suggestions for some edits and check out more of my Python for File Handling tutorials.