In this article we will explore how to rename files in a folder using Python.
Table of Contents
- Introduction
- Sample files
- Rename a single file using Python
- Rename multiple files using Python
- Conclusion
Introduction
When working with multiple files you may need to rename a particular file or multiple files.
This can be done manually, however if you are working with multiple files, or if your directory has many files, it can be a quiet time consuming task.
Using Python we can conveniently rename one or multiple files in a few lines of code.
In this tutorial we will work with the os module that provides a portable way of using operating system dependent functionality, so there is nothing additional to install.
Sample files
Here are the 3 sample files we will use in this tutorial:
and we will place them in the sample_files folder, so your directory with the code file looks like this:
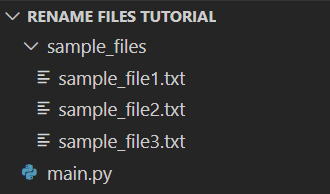
Rename a single file in a directory with Python
If the file is located in a folder, and you want to rename it without specifying the full path of the file, you can use the os module to navigate the folder and rename the file based on its relative path within that folder.
Let’s rename sample_file2.txt to sample_file2_new.txt:
#Import the required dependency
import os
#Define path to folder where file is located
folder_path = 'sample_files/'
#Define old file name
old_file_name = 'sample_file2.txt'
#Define new file name
new_file_name = 'sample_file2_new.txt'
#Get the relative full path to file
old_file_path = os.path.join(folder_path, old_file_name)
#Create the new path to file with new file name
new_file_path = os.path.join(folder_path, new_file_name)
#Rename the file
os.rename(old_file_path, new_file_path)
print(f'File renamed from {old_file_path} to {new_file_path}')
You should see the following message printed:
File renamed from sample_files/sample_file2.txt to sample_files/sample_file2_new.txt
and the file name in the directory will change:
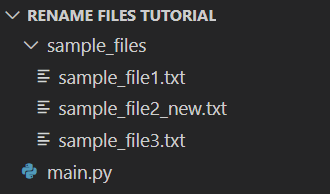
Rename multiple files in a directory with Python
In order to rename multiple files in a folder using Python, we will navigate to that folder, iterate over all the files in the directory, and rename the specific file we are looking for.
Note: In previous section we renamed one file from sample_file2.txt to sample_file2_new.txt, so let’s revert it back to the original file names:
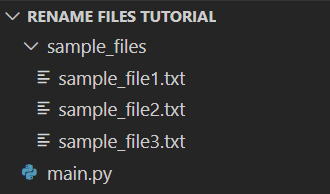
Let’s rename sample_file1.txt and sample_file3.txt to sample_file1_new.txt, and sample_file3_new.txt:
#Import the required dependency
import os
#Define path to folder where file is located
folder_path = 'sample_files/'
#Map old file names to new file names
file_rename_mapping = {
'sample_file1.txt': 'sample_file1_new.txt',
'sample_file3.txt': 'sample_file3_new.txt',
}
# Iterate through the files and rename them
for old_file_name, new_file_name in file_rename_mapping.items():
#Get the relative full path to file
old_file_path = os.path.join(folder_path, old_file_name)
#Create the new path to file with new file name
new_file_path = os.path.join(folder_path, new_file_name)
#Rename the file
os.rename(old_file_path, new_file_path)
print(f'File renamed from {old_file_path} to {new_file_path}')
and the file names in the directory will change:

Conclusion
In this article explored how to rename files in a folder using Python and os module.
Feel free to leave comments below if you have any questions or have suggestions for some edits and check out more of my Python for File Handling tutorials.