In this article we will explore how to manage environment variables using dotenv module in Python.
Table of Contents
- Introduction
- Create .env file
- Load environment variables from .env file
- Manage multiple environments with .env files
- Conclusion
Introduction
In the introductory article about environment variables in Python we discussed how to get and set environment variables manually using the os module.
As your Python project grows, you may be using more environment variables, or you might want to set the environment variables when the code is executed.
Instead of manually setting these environment variables, or hard coding them into the code, or using command line arguments to set the environment variables, you can create a .env file and use functionality from python-dotenv module to load them into your Python environment at runtime.
To continue following this tutorial we will need the following Python libraries: os and python-dotenv.
The os module is already built-in in Python, so we only need to install the python-dotenv module.
pip install python-dotenv
Create .env file
The first step is to create a .env file in the root directory of the project.
This file will contain the key-value pairs of the environment variables we would like to set for this project.
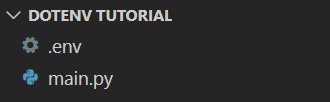
For this project, let’s create a TEST_USER and TEST_PASSWORD environment variables with some values, so your .env file should contain the following key-value pairs:
TEST_USER=user1
TEST_PASSWORD=12345
and look like this:
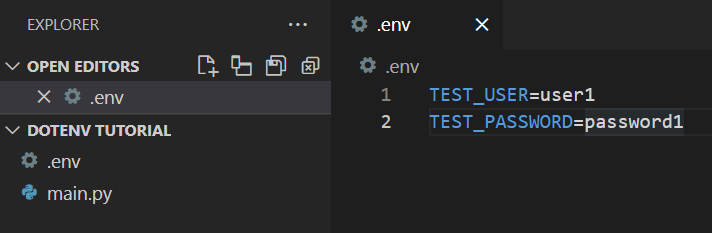
Next, we will see how to load these variables into the Python environment for the current session!
Load environment variables from .env file
Once we created the .env file with the environment variables we need for the project, the next step is to load them into the current Python environment.
The load_dotenv() function from dotenv module loads the environment variables from the .env file into the Python environment.
It reads the .env file and sets the environment variables specified in the file.
Once this step is completed, we can easily access these environment variables using os module’s functionality os.environ:
#Import the required dependencies
import os
from dotenv import load_dotenv
#Load environment variables from .env file
load_dotenv()
#Get specific environment variables
user_var = os.environ['TEST_USER']
pwd_var = os.environ['TEST_PASSWORD']
#Print environment variables
print(user_var)
print(pwd_var)
and you should get:
user1
12345
which are the environment variables’ values specified in the .env file.
Manage multiple environments with .env files
This section is for more advanced users working with multiple environments of their Python applications such as testing, development, and production.
In order to manage multiple environments with .env files, you will need to create a .env file for each of the environments, and store the required environment variables in them.
For example, let’s say you have 3 environments:
- Testing
- Development
- Production
You will need to create a .env file for each of them:
- .env.testing
- .env.development
- .env.production
so your root project folder will look like this:
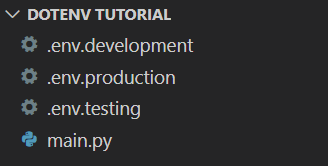
Here are the sample contents of each of the .env files:
.env.testing
API_KEY=test_api_12345
SECRET_KEY=test_12345
.env.development
API_KEY=dev_api_12345
SECRET_KEY=dev_12345
.env.production
API_KEY=prod_api_12345
SECRET_KEY=prod_12345
Now, let’s say the current environment of the project is testing, so we would like to access the environment variables from the appropriate .env file (which is .env.testing in this case):
#Import the required dependencies
import os
from dotenv import load_dotenv
#Set the current environment
env = 'testing'
#Load the appropriate .env file
if env == 'testing':
load_dotenv('.env.testing')
elif env == 'development':
load_dotenv('.env.development')
elif env == 'production':
load_dotenv('.env.production')
#Get specific environment variables
api_key = os.environ['API_KEY']
secret_key = os.environ['SECRET_KEY']
#Print environment variables
print(api_key)
print(secret_key)
and you should get:
test_api_12345
test_12345
Conclusion
In this article we explored how to manage environment variables using .env file and dotenv module in Python.
python-dotenv can be a very useful tool for managing environment variables and configuration options in your Python projects when you work with multiple environment variables, sensitive information, and want to stay away from hard coding these values into your code.
Feel free to leave comments below if you have any questions or have suggestions for some edits and check out more of my Python Programming tutorials.