In this article we will explore how to build a currency converter program in Python using ExchangeRate-API.
Table of Contents
Introduction
In this is a simple project we will use an exchange rate API to query the currency exchange rate data and use Python to build a program to display the results.
This tutorial is perfect for beginners that are starting to work on some simple Python projects.
To continue following this tutorial we will need the following Python library: requests.
pip install requests
Create API key
In this tutorial we will be working with ExchangeRate-API, one of the easiest to use and most reliable currency exchange rates APIs available.
In order to create your FREE API key, simply go to their homepage and enter your email in the form shown below and click “Get Free Key!”:

You will be prompted to choose a password and then you will get your API key:

In about 30 seconds you will get an email confirmation with a link to activate your account. Go ahead and click the activation link and you will be redirected to the dashboard:
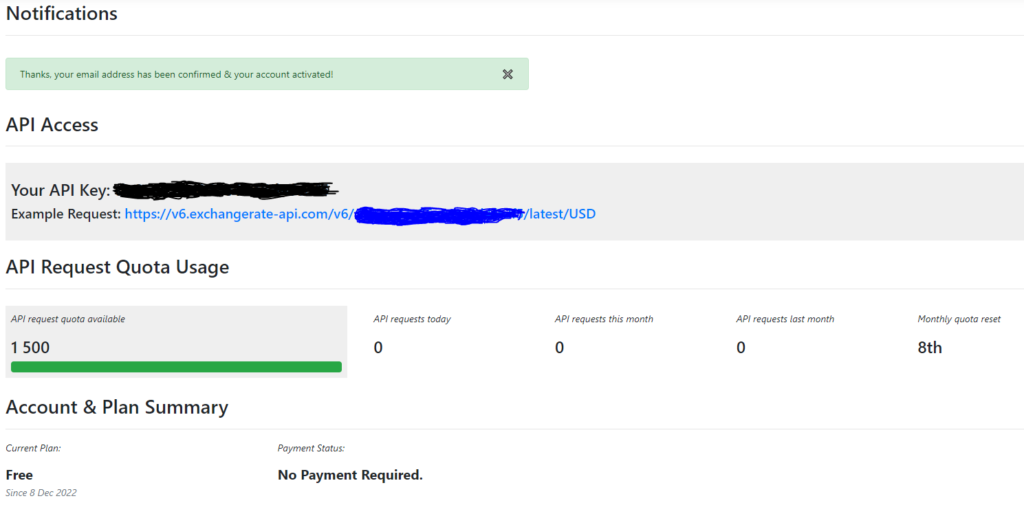
You will see an API key generated for you.
Create a credentials.py file in your project directory with the following code:
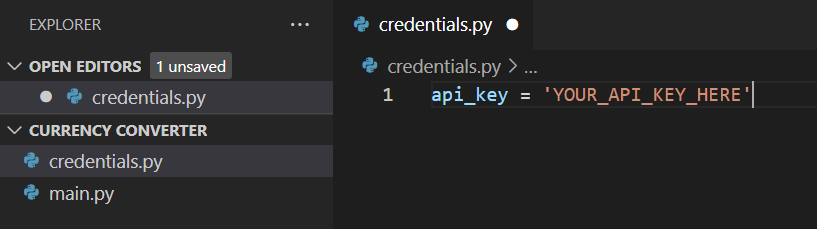
Now you have the exchange rate API key and we can continue to build the currency converter program in Python!
Build currency converter program in Python
Let’s start with importing the required dependencies. We will need to import the requests module as well as the api_key from credentials.py:
#Import the required dependencies
import requests
from credentials import api_key
Then we will define a function that asks the user to input the currencies between which they would like to do the conversion as well as the amount:
#Ask user to input currencies for conversion and the amount
def get_user_input():
curr_from = input('Please enter the currency from which you would like to convert: ').upper()
curr_to = input('Please enter the currency to which you would like to convert: ').upper()
amount = int(input('Please the amount you would like to convert: '))
return curr_from, curr_to, amount
Next, let’s find the URL for the GET request for the pair conversion. It will look like this:
https://v6.exchangerate-api.com/v6/YOUR-API-KEY/pair/EUR/GBP/AMOUNT
and the GET request will return a JSON file containing the result of the currency conversion similar to the one below:
{
"result": "success",
"documentation": "https://www.exchangerate-api.com/docs",
"terms_of_use": "https://www.exchangerate-api.com/terms",
"time_last_update_unix": 1585267200,
"time_last_update_utc": "Fri, 27 Mar 2020 00:00:00 +0000",
"time_next_update_unix": 1585270800,
"time_next_update_utc": "Sat, 28 Mar 2020 01:00:00 +0000",
"base_code": "EUR",
"target_code": "GBP",
"conversion_rate": 0.8412,
"conversion_result": 5.8884
}
Once we see the structure of the URL for the GET request, we can easily create a function that will perform the GET request and return the converted amount:
#Get converted amount
def get_converted_amount(curr_from, curr_to, amount):
#Create a URL for pair converstion
url = f'https://v6.exchangerate-api.com/v6/{api_key}/pair/{curr_from}/{curr_to}/{amount}'
#Parse the result as JSON
data = requests.get(url).json()
#Extract the converted amount
converted_amount = data['conversion_result']
return converted_amount
And finally we will add the code executor part:
if __name__ == '__main__':
curr_from, curr_to, amount = get_user_input()
converted_amount = get_converted_amount(curr_from, curr_to, amount)
print(f'{amount} {curr_from} = {converted_amount} {curr_to}')
Complete code for currency converter
#Import the required dependencies
import requests
from credentials import api_key
#Ask user to input currencies for conversion and the amount
def get_user_input():
curr_from = input('Please enter the currency from which you would like to convert: ').upper()
curr_to = input('Please enter the currency to which you would like to convert: ').upper()
amount = int(input('Please the amount you would like to convert: '))
return curr_from, curr_to, amount
#Get converted amount
def get_converted_amount(curr_from, curr_to, amount):
#Create a URL for pair converstion
url = f'https://v6.exchangerate-api.com/v6/{api_key}/pair/{curr_from}/{curr_to}/{amount}'
#Parse the result as JSON
data = requests.get(url).json()
#Extract the converted amount
converted_amount = data['conversion_result']
return converted_amount
if __name__ == '__main__':
curr_from, curr_to, amount = get_user_input()
converted_amount = get_converted_amount(curr_from, curr_to, amount)
print(f'{amount} {curr_from} = {converted_amount} {curr_to}')
Conclusion
In this article we covered how you can build a currency converter program in Python using ExchangeRate-API. I also encourage you to check out my other posts on Python Programming.
Feel free to leave comments below if you have any questions or have suggestions for some edits.