In this tutorial we will explore how to convert CSV file to HTML table using Python.
Table of Contents
Introduction
Converting CSV to HTML tables is useful for making some reports that you would like to share on the web.
Instead of uploading the whole CSV file and having the end user download it and look through it, we can simply convert a CSV into an HTML table by generating an HTML file which can be opened by the user’s default browser.
To continue following this tutorial we will need the following Python library: pandas.
If you don’t have it installed, please open “Command Prompt” (on Windows) and install it using the following code:
pip install pandas
Sample CSV file
Here is the CSV file we will use in this tutorial:
which has the following data:
student_id | first_name | last_name | grade |
1221 | John | Smith | 86 |
1222 | Erica | Write | 92 |
1223 | Will | Erickson | 74 |
1224 | Madeline | Berg | 82 |
1225 | Mike | Ellis | 79 |
Convert CSV to HTML Table using Python
Using pandas library we can convert CSV to HTML table in a few lines of code:
#Import the required dependency
import pandas as pd
#Read CSV file
df = pd.read_csv('grades.csv')
#Export as HTML file
df.to_html('grades.html')
and you should see grades.html generated in the same folder:
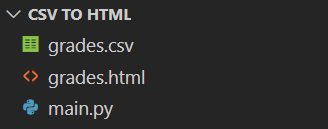
with HTML code that looks like this:
<table border="1" class="dataframe">
<thead>
<tr style="text-align: right;">
<th></th>
<th>student_id</th>
<th>first_name</th>
<th>last_name</th>
<th>grade</th>
</tr>
</thead>
<tbody>
<tr>
<th>0</th>
<td>1221</td>
<td>John</td>
<td>Smith</td>
<td>86</td>
</tr>
<tr>
<th>1</th>
<td>1222</td>
<td>Erica</td>
<td>Write</td>
<td>92</td>
</tr>
<tr>
<th>2</th>
<td>1223</td>
<td>Will</td>
<td>Erickson</td>
<td>74</td>
</tr>
<tr>
<th>3</th>
<td>1224</td>
<td>Madeline</td>
<td>Berg</td>
<td>82</td>
</tr>
<tr>
<th>4</th>
<td>1225</td>
<td>Mike</td>
<td>Ellis</td>
<td>79</td>
</tr>
</tbody>
</table>
File attached below:
Opening this file with your default browser should show the data in a table format:
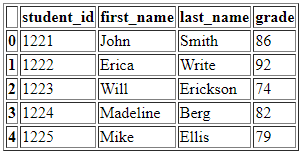
Conclusion
In this article we explored how to convert CSV file to HTML table using Python and pandas.
Feel free to leave comments below if you have any questions or have suggestions for some edits and check out more of my Python Programming tutorials.